Mastering the Python Crypto Trading Bot: A Comprehensive Guide
In recent years, cryptocurrency trading has gained immense popularity among both seasoned investors and casual traders. As the market continues to evolve, the need for automated trading tools, specifically trading bots, has surged. This article aims to provide an in-depth exploration of creating a Python crypto trading bot, highlighting crucial components, strategies, and my own insights on the subject.
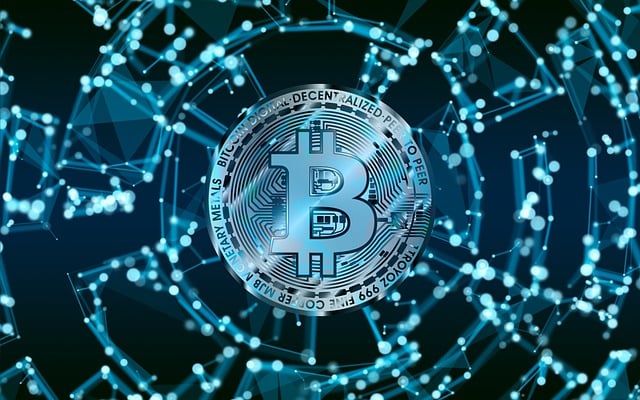
Understanding Crypto Trading Bots
Before diving into the technicalities, it’s essential to grasp what crypto trading bots are and how they function. In essence, a trading bot is a software application that interacts with cryptocurrency exchanges to automate buying and selling activities. Bots can execute orders at speeds and frequencies unmanageable for humans, making them invaluable in a volatile market.
Types of Trading Bots
There is a myriad of trading bots available, each catering to different trading strategies and risk appetites. Here are some of the most common types:
- Market Making Bots: These bots provide liquidity to the market by placing buy and sell orders at varying prices.
- Arbitrage Bots: These bots exploit price discrepancies between different exchanges to ensure profit.
- Trend Following Bots: These bots analyze market trends and execute trades based on identified patterns.
- Mean Reversion Bots: These tools predict price corrections and profit from the reversion to mean price levels.
My Opinion on Bot Types
From my perspective, the choice of trading bot heavily relies on an individual’s trading philosophy. Trend following bots resonate with many traders inspired by the adage, "the trend is your friend." Meanwhile, those who prefer to capitalize on market inefficiencies may lean towards arbitrage bots. A well-informed choice can substantially enhance the trading experience.
The Basics of Python for Trading Bots
Python has garnered significant attention within the trading community due to its versatility and simplicity. Whether you're a novice programmer or a seasoned developer, Python's robust libraries facilitate the creation and deployment of trading bots.
Essential Libraries
Several libraries stand out in the development of a trading bot:
- Pandas: A powerful data manipulation library that allows for extensive analysis of trading data.
- NumPy: Ideal for performing numerical computations, which is crucial for executing trading algorithms.
- ccxt: A library that provides a unified way to connect with different cryptocurrency exchanges.
- Matplotlib: This library is essential for visualizing data and backtesting trading strategies.
Why I Recommend Python
In my opinion, Python’s readability and supportive community make it an excellent choice for both beginners and experienced developers. I believe that having a programming language that promotes experimentation without overwhelming complexity is invaluable, especially in the fast-paced world of crypto trading.
Designing Your Crypto Trading Bot
Setting Up Your Environment
Before we can start coding, it’s crucial to set up your development environment properly. You need to ensure that you have the necessary libraries installed. You can do so using pip:
pip install pandas numpy ccxt matplotlib
Connecting to Crypto Exchanges
To trade, your bot needs to connect with cryptocurrency exchanges. The ccxt library allows you to easily integrate with multiple exchanges. Below is a simple example of connecting to Binance:
import ccxt
exchange = ccxt.binance({
'apiKey': 'YOUR_API_KEY',
'secret': 'YOUR_API_SECRET',
})
Examining Market Data
The ability to quickly analyze market data is vital for any trading strategy. Here, you can leverage Python’s Pandas library to structure and analyze the data obtained:
def fetch_data(symbol, timeframe='1m', limit=100):
bars = exchange.fetch_ohlcv(symbol, timeframe=timeframe, limit=limit)
return pd.DataFrame(bars, columns=['timestamp', 'open', 'high', 'low', 'close', 'volume'])
The Value of Data Analysis
I contend that data analysis is perhaps one of the most crucial aspects of trading bot development. Making data-driven decisions rather than emotional ones can inherently improve your trading outcomes. In the chaos of crypto markets, statistics are your best friends.
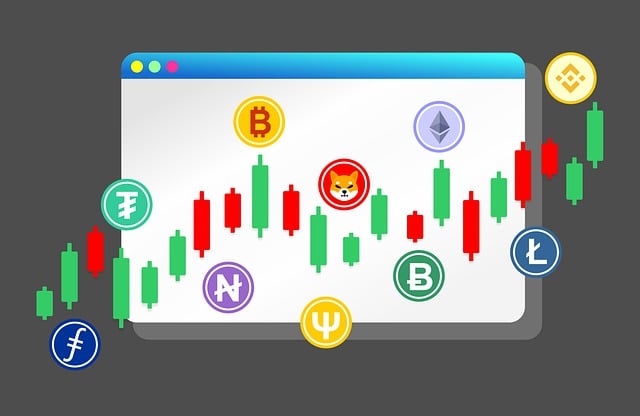
Implementing Trading Strategies
When building a crypto trading bot, it's imperative to determine your strategy based on market analysis. Here are a few widely used strategies:
Strategy 1: Moving Average Crossover
The moving average crossover strategy is a popular method for identifying potential buy and sell signals.
def moving_average_crossover(symbol):
data = fetch_data(symbol)
short_ma = data['close'].rolling(window=5).mean()
long_ma = data['close'].rolling(window=20).mean()
# Buy signal
if short_ma.iloc[-1] > long_ma.iloc[-1]:
return 'Buy'
# Sell signal
else:
return 'Sell'
Why This Strategy Appeals to Me
I find moving average crossover appealing due to its simplicity and effectiveness in capturing trends. It lays the groundwork for developing more complex strategies while allowing room for expansion.
Strategy 2: RSI (Relative Strength Index)
The RSI strategy involves tracking momentum and identifying overbought or oversold conditions.
def rsi(symbol, period=14):
data = fetch_data(symbol)
delta = data['close'].diff()
gain = (delta.where(delta > 0, 0)).rolling(window=period).mean()
loss = (-delta.where(delta < 0, 0)).rolling(window=period).mean()
rs = gain / loss
return 100 - (100 / (1 + rs))
The Importance of RSI in My Trading Approach
In my opinion, the RSI is a powerful indicator for assessing market conditions. It offers a more nuanced view of market sentiment compared to price action alone, enabling traders to make well-informed decisions.
Backtesting Your Strategy
Once you've developed your strategy, backtesting is crucial to ascertain its viability. Backtesting allows you to simulate trades based on historical data, providing insights into potential performance.
Creating a Backtesting Framework
def backtest(strategy, symbol, start_date, end_date):
# Suppose you have a function to fetch historical data
data = fetch_historical_data(symbol, start_date, end_date)
# Apply the chosen strategy
signals = strategy(data)
return signals
Why Backtesting is Indispensable
In my view, neglecting to backtest a strategy is one of the most significant mistakes traders make. A carefully constructed backtesting framework can highlight weaknesses and enhance strategy robustness before risking actual capital.
Live Trading and Risk Management
Transitioning to Live Trading
Moving from backtesting to live trading can be daunting. It is vital to start with a demo account or small capital, allowing the bot to operate in real conditions without significant financial risk.
Implementing Risk Management Strategies
Effective risk management cannot be overstated. Here are a few key practices:
- Setting stop-loss orders to limit potential losses.
- Diversifying investments across multiple cryptocurrencies.
- Regularly evaluating trading performance and making necessary adjustments.
My Approach to Risk Management
In my opinion, a trader’s success hinges significantly on how well they manage risk. I firmly believe that protecting your capital is just as crucial as implementing winning strategies. Without a solid risk management plan, even the best strategies can lead to devastating losses.
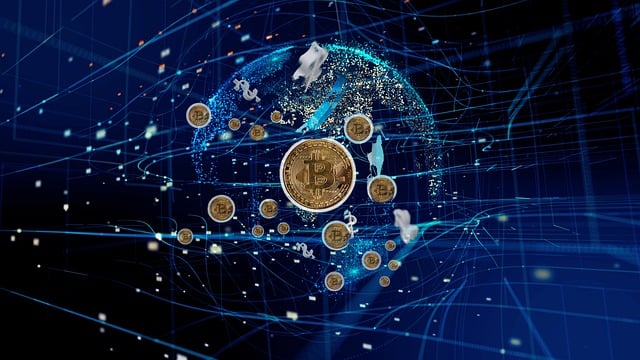
Conclusion: The Future of Trading Bots
In conclusion, the world of cryptocurrency is ever-evolving, and Python trading bots offer an opportunity to navigate this dynamic environment effectively. While the creation of a trading bot may seem daunting initially, the reward of automated trading amidst market volatility is well worth the investment of time and effort.
Becoming proficient in bot development requires practice, continual learning, and adaptation to new trends and technologies. As for myself, I am continually intrigued by the advancements in the trading bot landscape, and I believe that the future will see even more sophisticated tools that harness AI and machine learning to generate smarter trading strategies.
Ultimately, whether you're a novice trader or a seasoned veteran, the key takeaway is simple: a well-designed bot can streamline trading processes, mitigate emotional trading pitfalls, and yield better long-term results. Happy trading!