Comprehensive Guide to Building a Python Binance Trading Bot
Creating a trading bot on the Binance platform using Python is an exhilarating venture. Not only does it help automate trading processes, but it also allows traders to explore new strategies and make data-driven decisions. In this detailed tutorial, I'll walk you through the steps to create your own Binance trading bot, offering insights, opinions, and best practices along the way.
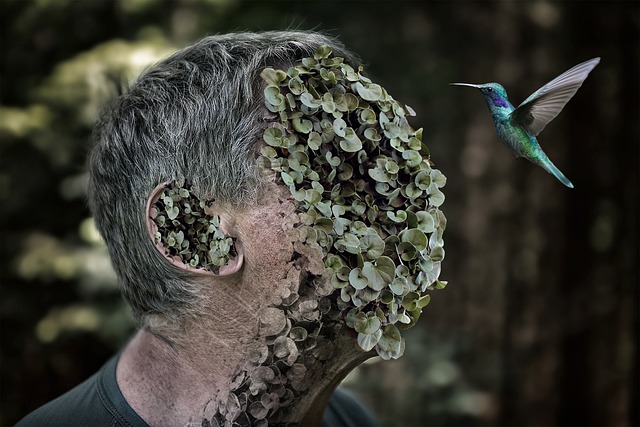
Understanding the Binance API
Before diving into the code, it’s crucial to comprehend what the Binance API is and how it operates. The Binance API enables developers to interface programmatically with the Binance trading platform, accessing various functionalities such as market data, trade history, and account management.
Setting Up Your Binance Account
Your first step will be to set up a Binance account. If you already have an account, make sure you've completed the necessary verification procedures.
Create API Keys
Once your account is set up, navigate to the API management section. Here, you can create your API key. This key is crucial for connecting your Python script to the Binance API. Ensure to:
- Save your API key and secret securely.
- Restrict API key access to specific IP addresses for security.
Preparing Your Development Environment
Before writing your bot, you'll need the right tools. I personally recommend using a virtual environment to manage dependencies effectively.
Installing Required Libraries
Open your terminal or command prompt and type the following commands to set up your virtual environment and install the necessary libraries:
python -m venv binance-bot-env
source binance-bot-env/bin/activate # On Windows use: binance-bot-env\Scripts\activate
pip install python-binance pandas
Here, we're using the python-binance
package to interact with the Binance API and pandas
for handling data analysis.
Creating a Basic Binance Bot
Now comes the fun part! Let’s dive into coding your bot. The objective here is to make a simple bot that fetches market data and executes trades based on predefined conditions.
Connecting to the API
Begin by creating a new Python file and importing the necessary libraries:
from binance.client import Client
import pandas as pd
import time
Next, you’ll need to set up your API keys:
API_KEY = 'YOUR_API_KEY'
API_SECRET = 'YOUR_API_SECRET'
client = Client(API_KEY, API_SECRET)
Replace YOUR_API_KEY
and YOUR_API_SECRET
with your actual keys. This will create a client that you can use to interact with the Binance API.
Fetching Market Data
Now let's define a function to fetch historical candlestick data:
def fetch_historical_data(symbol, interval, lookback):
klines = client.get_historical_klines(symbol, interval, lookback)
return pd.DataFrame(klines, columns=['Open time', 'Open', 'High', 'Low', 'Close', 'Volume', 'Close time', 'Quote asset volume', 'Number of trades', 'Taker buy base asset volume', 'Taker buy quote asset volume', 'Ignore'])
This function takes the trading symbol
, the interval
of candlesticks (e.g., '1h' for one hour), and lookback
period (such as '1 day') to provide historical market data.
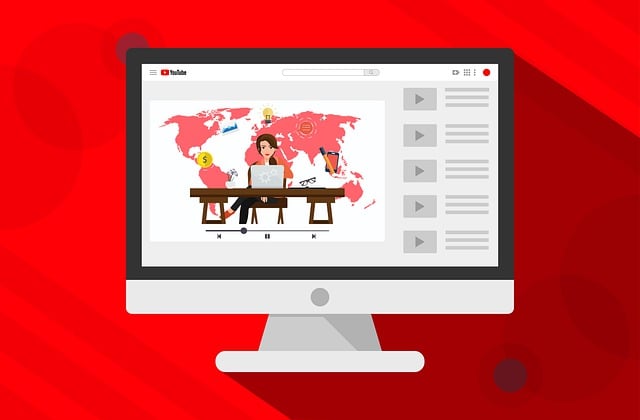
Implementing Trading Logic
Once you have the data, you need to implement your trading strategy. For simplicity, let's create a basic moving average crossover strategy.
Defining the Trading Strategy
You can calculate the moving averages of the closing prices like this:
def moving_average_crossover(symbol):
df = fetch_historical_data(symbol, Client.KLINE_INTERVAL_1HOUR, "1 day ago UTC")
df['Close'] = df['Close'].astype(float)
df['MA20'] = df['Close'].rolling(window=20).mean()
df['MA50'] = df['Close'].rolling(window=50).mean()
if df['MA20'].iloc[-1] > df['MA50'].iloc[-1]:
return "BUY"
elif df['MA20'].iloc[-1] < df['MA50'].iloc[-1]:
return "SELL"
else:
return "HOLD"
In this code snippet, we calculate the 20-period and 50-period moving averages and return the appropriate signal. While this strategy is simple, it's important to note that no trading strategy is foolproof. It requires backtesting and adjustments based on market behavior.
Executing Trades
If the trading logic decides to execute a buy or sell order, here’s how you can implement the trade function:
def place_order(symbol, side, quantity):
try:
order = client.order_market(
symbol=symbol,
side=side,
quantity=quantity
)
return order
except Exception as e:
print(f"An error occurred: {str(e)}")
Integrating Trading Logic with Order Execution
You can now integrate your trading logic with the order execution function. This will continuously check the market every hour and execute trades based on the signals generated from the moving average strategy:
while True:
action = moving_average_crossover('BTCUSDT')
if action == "BUY":
print("Placing buy order...")
place_order('BTCUSDT', 'BUY', 0.001) # Adjust quantity as needed
elif action == "SELL":
print("Placing sell order...")
place_order('BTCUSDT', 'SELL', 0.001)
time.sleep(3600) # Wait for an hour before checking again
This loop will run indefinitely, checking the market every hour. However, I would like to emphasize the importance of implementing risk management strategies and not using a significant amount of capital, especially while testing your bot.
Best Practices and Final Notes
Building a bot is just the beginning. Here are some best practices I’ve gathered over time:
Backtesting Your Strategy
Before committing real funds to your bot, ensure to backtest your strategy using historical data. This practice allows you to evaluate its performance under various market conditions.
Implementing Stop Loss/Take Profit Mechanisms
Risk management is crucial. Incorporate functionalities for stop-loss and take-profit orders to minimize potential losses and secure profits, respectively.
Continuous Monitoring
Even though your bot is automated, daily or regular monitoring of its performance and adapting your strategy to market changes is essential.
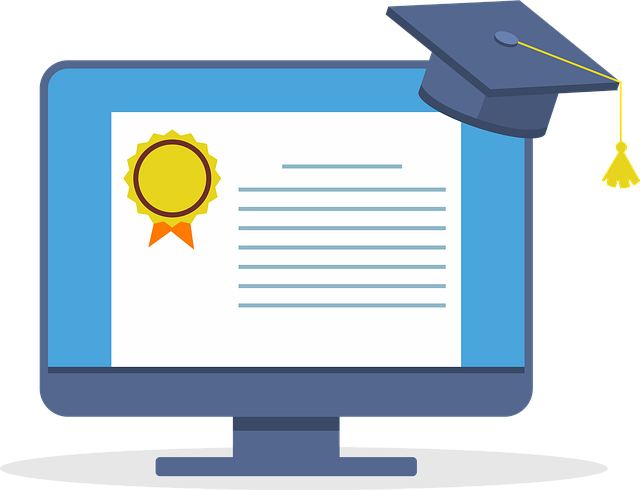
Conclusion
Creating a Python Binance trading bot can be a fulfilling project that opens doors to automated trading. While the example provided here is simplistic, it serves as a foundation. I feel that experimenting with different strategies, continuing to learn about algorithmic trading, and staying updated on market trends are keys to growing as both a programmer and a trader. Remember, the crypto market is highly volatile, and no automated system is guaranteed to succeed. Always trade wisely and only invest what you can afford to lose.
I hope this tutorial serves as an equally educational and inspiring guide for your trading bot journey. Good luck!