The Comprehensive Guide to Creating a Binance Trading Bot
In the rapidly evolving world of cryptocurrency trading, automated trading bots have emerged as a powerful tool for traders and investors alike. As cryptocurrency prices fluctuate wildly, having a reliable trading bot can help maximize profits while minimizing risks. Among the myriad of trading platforms, Binance stands out as one of the premier options for trading various cryptocurrencies. This article aims to provide a detailed overview of creating a Binance trading bot, discussing everything from the basics of trading bots to the intricacies of programming one for the Binance platform.
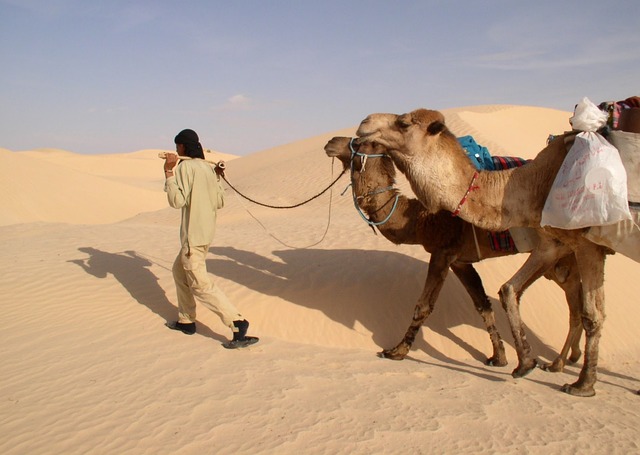
Understanding Binance and Its API
Before diving into the nitty-gritty of building a trading bot, it is crucial to understand what Binance is and how its API works. Binance is a global cryptocurrency exchange that offers a platform for trading a wide range of cryptocurrencies. With millions of users and billions in daily trading volume, Binance provides both experienced traders and novices the tools they need to engage in crypto trading.
What is Binance API?
The Binance API allows developers to connect their applications to the Binance platform. This API offers a plethora of functionalities, enabling the automation of trading operations, retrieval of market data, and monitoring of account details. The Binance API is crucial for anyone looking to develop a trading bot, as it provides the necessary endpoints to perform trades programmatically.
Key Features of Binance API
- Real-time market data retrieval
- Order management (placing and cancelling orders)
- Account information, including balances and transaction history
- WebSocket streams for real-time price updates
Setting Up Your Development Environment
Now that we have a basic understanding of Binance and its API, the next step is to set up a development environment for creating your bot. The choice of programming language can significantly impact your bot's performance and ease of development. Typically, languages like Python, JavaScript, and Java are popular options.
Installing Required Libraries
For this tutorial, we will use Python due to its simplicity and extensive library support. To begin, you will need to install the following libraries:
- Requests: for making API requests
- Pandas: for data analysis
- NumPy: for numerical calculations
- TA-Lib or similar: for technical indicators
You can install these libraries using pip:
pip install requests pandas numpy TA-Lib
Creating a Binance Account and API Keys
To utilize the Binance API, you must create a Binance account and generate API keys. Here’s how to do that:
Step 1: Register on Binance
If you do not already possess a Binance account, visit the official Binance website and register. Ensure that you complete any verification processes required by the platform.
Step 2: Generate API Keys
Once you have registered, navigate to the API Management section of your account settings. Here, you can create a new API key that will provide access to your account through the API. Make sure to store your Secret Key securely, as it will not be displayed again after creation.
Important Security Measures
When working with your API keys, it is vital to follow robust security protocols. Here are some tips:
- Never share your API keys with anyone.
- Restrict API key access to specific IP addresses if possible.
- Use API keys with limited permissions (e.g., only allow trading and not withdrawals).
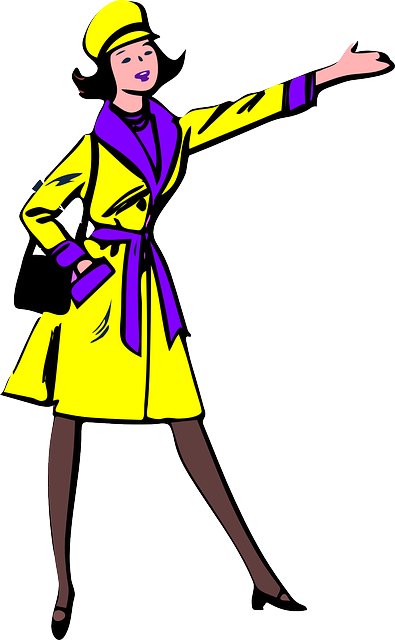
Building the Trading Bot
With your development environment set up and API keys generated, we can now move on to coding the actual trading bot. Below, we will outline a simple trading strategy for illustration.
Choosing a Trading Strategy
The effectiveness of a trading bot largely relies on the underlying strategy it employs. Common strategies include:
- Arbitrage: Taking advantage of price differences between exchanges.
- Market Making: Providing liquidity by placing buy and sell orders.
- Trend Following: Following market trends using indicators.
For this article, we will develop a simple trend-following bot that utilizes moving averages for decision-making. The bot will buy when the short-term moving average crosses above the long-term moving average and sell when the opposite occurs.
Coding the Trading Bot
Here is a simplified version of how the trading bot can be structured:
import requests
import pandas as pd
import time
# Set your API keys
API_KEY = 'your_api_key'
API_SECRET = 'your_api_secret'
BASE_URL = 'https://api.binance.com'
# Function to get historical price data
def get_historical_data(symbol, interval, limit):
url = f"{BASE_URL}/api/v3/klines?symbol={symbol}&interval={interval}&limit={limit}"
response = requests.get(url)
data = response.json()
return pd.DataFrame(data)
# Function to calculate moving averages
def calculate_moving_averages(data, short_window, long_window):
data['Short_MA'] = data['close'].astype(float).rolling(window=short_window).mean()
data['Long_MA'] = data['close'].astype(float).rolling(window=long_window).mean()
return data
# Function to execute trades
def place_order(symbol, side, quantity):
url = f"{BASE_URL}/api/v3/order"
order_data = {
'symbol': symbol,
'side': side,
'type': 'MARKET',
'quantity': quantity,
'timestamp': int(time.time() * 1000)
}
headers = {'X-MBX-APIKEY': API_KEY}
response = requests.post(url, headers=headers, data=order_data)
return response.json()
symbol = "BTCUSDT"
data = get_historical_data(symbol, '1h', 100)
data = calculate_moving_averages(data, short_window=9, long_window=21)
if data['Short_MA'].iloc[-1] > data['Long_MA'].iloc[-1]:
place_order(symbol, 'BUY', quantity=0.001)
elif data['Short_MA'].iloc[-1] < data['Long_MA'].iloc[-1]:
place_order(symbol, 'SELL', quantity=0.001)
This code illustrates the fundamental structure of a trading bot. It retrieves historical price data, calculates moving averages, and places buy or sell orders based on crossovers of these moving averages.
Backtesting the Strategy
Before deploying a trading bot in the live market, it is critical to backtest the strategy against historical price data. This process involves running the trading algorithm using past market conditions to evaluate its performance.
Backtesting allows the trader to assess key metrics such as:
- Win rate
- Average profit/loss per trade
- Maximum drawdown
Tools like Backtrader or Zipline can be incredibly helpful for conducting backtests, providing a user-friendly interface for analyzing strategy performance.
Deploying the Bot
Once you have thoroughly backtested your trading bot and are satisfied with its performance, you can deploy it to trade with real money. However, there are several factors to consider before going live:
Monitoring and Maintenance
Automated trading does not mean 'set and forget.' Regular monitoring is essential to ensure that the bot is functioning as intended. Common tasks during this phase may include:
- Reviewing trade performance and making adjustments if necessary.
- Updating the bot based on changing market conditions.
- Staying informed about potential changes to the Binance API.
Risk Management
Implementing robust risk management strategies is imperative when using a trading bot. This may include:
- Setting a maximum loss limit for each trade.
- Diversifying your portfolio to spread risk.
- Regularly assessing the effectiveness of your trading strategies.
Conclusion
In conclusion, creating a Binance trading bot can be a rewarding endeavor for those looking to automate their trading strategies. While this article provides a foundational understanding and a simple example of a trading bot, the possibilities are vast, and traders can implement more complex strategies based on their analysis and market conditions.
That being said, always proceed with caution when trading in the volatile crypto market. Using a trading bot does not guarantee profits and carries risks. Therefore, thorough research, testing, and ongoing analysis are essential components of successful algorithmic trading.
As the world of automated trading continues to grow, there will always be new strategies, tools, and technologies to explore. Engaging with communities of developers and traders can also provide valuable insights and enhance your learning curve in this exciting field.
Ultimately, building and maintaining a Binance trading bot can be both a thrilling and educational journey in the realm of cryptocurrency trading.