func main() {
var randomBytes [8]byte
_, err := rand.Read(randomBytes[:])
if err != nil {
fmt.Println("Error:", err)
return
}
fmt.Println(randomBytes)
}
Golang crypto/rand is a powerful package that provides a secure source of random numbers. It can be used in various scenarios, such as cryptography, random key generation, and more. In this article, we explored the basics of using Golang crypto/rand to generate random numbers and integers. By leveraging this package, you can enhance the security and reliability of your Go applications.
References:
5. డబ్బు సంపాదించడానికి ఏ గేమ్స్ ను ఆడవాలి?
In addition to generating random bytes, the crypto/rand package also provides a function to generate random integers within a specified range. The 'Int' function from the 'Reader' type can be used for this purpose. Here's an example:
func main() {
randomInt, err := rand.Int(rand.Reader, big.NewInt(100))
if err != nil {
fmt.Println("Error:", err)
return
}
fmt.Println(randomInt)
}
Golang crypto/rand
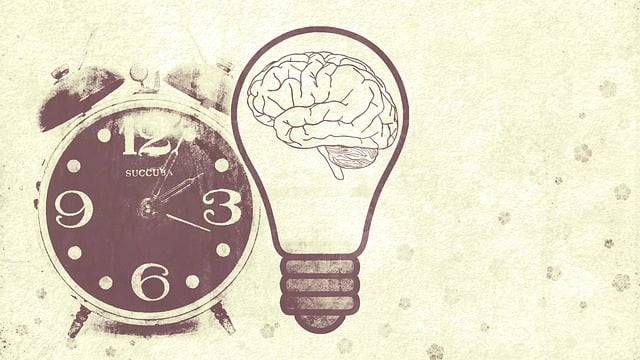
Introduction
Golang crypto/rand is a package in the Go programming language that provides a source of cryptographically secure random numbers. This package is commonly used in cryptography and other applications where randomness is required. In this article, we will explore the features and usage of Golang crypto/rand.
Importing crypto/rand
Article link: 5. డబ్బు సంపాదించడానికి ఏ గేమ్స్ ను ఆడవాలి?
Author: Crypto Trade Signals
In this example, we use the 'Int' function to generate a random integer between 0 and 100. The first argument to the 'Int' function is the random number generator source, which is set to 'rand.Reader'. The second argument is the upper bound (exclusive) of the generated random integer. We handle any errors that might occur and print the generated random integer.
Conclusion
To use the crypto/rand package in your Go program, you need to import it first. This can be done using the following import statement:
import "crypto/rand"
Generating random numbers
In this example, we declare a byte array of size 8 and then call the 'Read' function to fill it with random bytes. We handle any errors that might occur during the generation of random bytes. Finally, we print the generated random bytes.
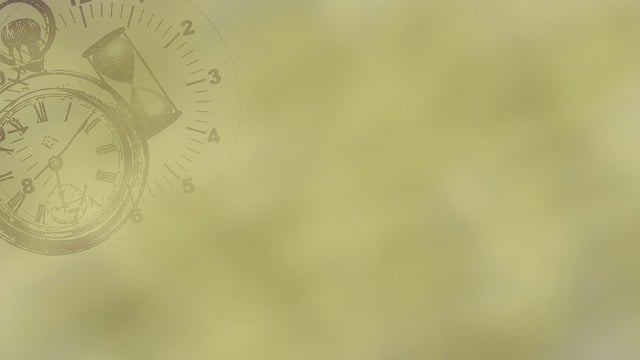