Python Binance Bot Tutorial: A Comprehensive Guide
In the ever-evolving world of cryptocurrency trading, automation has become essential for many traders seeking to maximize profit while minimizing effort. Among the various tools available, trading bots have surged in popularity, particularly when interfaced through platforms like Binance. This tutorial is designed to walk you through the process of creating a simple trading bot using Python, with step-by-step instructions and insightful tips to enhance your trading experience.
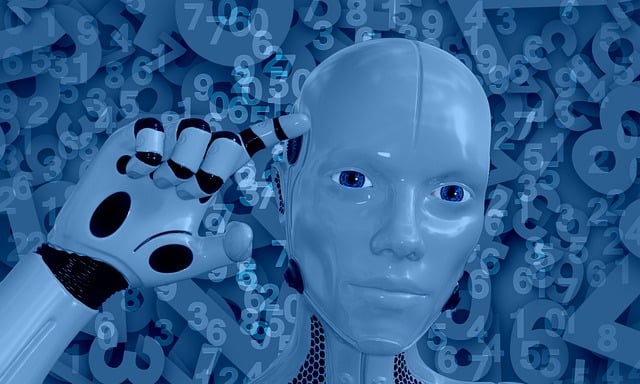
What is a Binance Trading Bot?
A Binance trading bot is a program that automates trading activities on the Binance exchange. By utilizing predefined strategies, such as market making or arbitrage, these bots can perform buy and sell operations at speeds and frequencies beyond human capability. This automation allows traders to take advantage of market fluctuations continuously, without being tethered to their screens.
Why Use a Trading Bot?
Using a trading bot provides several distinct advantages:
- Efficiency: Bots can operate 24/7 without requiring breaks. This allows traders to capitalize on market opportunities at any time.
- Speed: Bots can execute trades in milliseconds, which is crucial in a volatile market where prices can change rapidly.
- Emotion-Free Trading: Trading bots follow the technical and strategic parameters set by the user, eliminating emotional decision-making that can lead to losses.
Setting Up Your Python Environment
Before you can start coding your Binance trading bot, you need a working Python environment. Here’s how to set it up:
Step 1: Install Python
First, download and install Python from python.org. Make sure you add Python to your PATH during the installation process.
Step 2: Install Necessary Libraries
Once Python is successfully installed, you need to install the Binance API wrapper and other necessary libraries. Open your command prompt (or terminal) and run the following commands:
pip install python-binance
pip install pandas
pip install numpy
pip install matplotlib
Creating Your First Binance Trading Bot
With your environment set up, it’s time to start coding. Below is a basic example of a trading bot that uses the Binance API to execute trades.
Step 1: Import Libraries
import os
from binance.client import Client
import pandas as pd
import numpy as np
Step 2: API Authentication
To interact with the Binance API, you’ll need an API key and secret. You can obtain these from your Binance account settings under API Management. Once you have them, store them in environment variables for security:
API_KEY = os.environ.get('BINANCE_API_KEY')
API_SECRET = os.environ.get('BINANCE_API_SECRET')
client = Client(API_KEY, API_SECRET)
Step 3: Fetch Historical Data
You will need historical data to formulate your trading strategy. Here’s how to fetch it:
def get_historical_data(symbol, interval, start_time):
df = pd.DataFrame(client.get_historical_klines(symbol, interval, start_time))
df.columns = ["Open Time", "Open", "High", "Low", "Close", "Volume", "Close Time",
"Quote Asset Volume", "Number of Trades", "Taker Buy Base Volume",
"Taker Buy Quote Volume", "Ignore"]
return df
Step 4: Define Your Trading Strategy
Let’s define a simple moving average crossover strategy as an example:
def simple_moving_average_crossover(symbol):
data = get_historical_data(symbol, Client.KLINE_INTERVAL_1MINUTE, "1 day ago UTC")
data['Close'] = data['Close'].astype(float)
data['SMA_short'] = data['Close'].rolling(window=5).mean()
data['SMA_long'] = data['Close'].rolling(window=20).mean()
if data['SMA_short'].iloc[-1] > data['SMA_long'].iloc[-1]:
return "BUY"
elif data['SMA_short'].iloc[-1] < data['SMA_long'].iloc[-1]:
return "SELL"
else:
return "HOLD"
Step 5: Execute Trades
Finally, implement the execution of trades based on your trading signals:
def execute_trade(signal, symbol, quantity):
if signal == "BUY":
order = client.order_market_buy(symbol=symbol, quantity=quantity)
elif signal == "SELL":
order = client.order_market_sell(symbol=symbol, quantity=quantity)
return order
Step 6: Putting It All Together
Now that you have defined your trading logic, you can call these functions in a loop to keep checking for trade signals:
while True:
signal = simple_moving_average_crossover("BTCUSDT")
execute_trade(signal, "BTCUSDT", 0.01)
time.sleep(60) # Wait for one minute before checking again
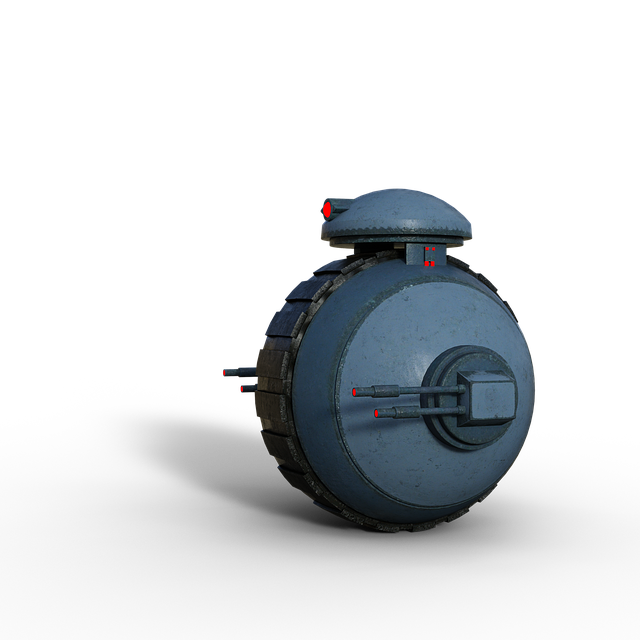
Advanced Configurations
Now that you have a basic trading bot, it’s time to consider more advanced configurations. These enhancements can help improve your trading strategy tremendously.
Implementing Risk Management
Risk management is crucial for any trading strategy. Consider implementing features like:
- Stop-Loss Orders: Automatically sell an asset once it reaches a certain price.
- Take-Profit Orders: Secure profits by selling once a target price is achieved.
Backtesting Your Strategy
Before deploying your bot, backtest your trading strategy using historical data to assess its effectiveness. You can use libraries such as Backtrader or Zipline for this purpose.
Monitoring and Alerts
For effective operations, set up notifications through Telegram or email to keep you updated on your bot's performance. This way, you’ll be informed about significant trades or failures.
Additional Resources
While this tutorial provides an excellent introduction, cryptocurrency trading is complex and dynamic. Here are additional articles worth exploring:
- Understanding Crypto MEV Bots: Navigating the New Frontier of Cryptocurrency Trading: This article delves into Maximum Extractable Value (MEV) bots, focusing on how advanced algorithms can identify profitable trading opportunities and strategies.
- Binance Trading Bot C#: Enhancing Your Trading Experience: If you're a C# developer, this article provides insights on building effective trading bots using C#, allowing for robust automated trading strategies.
- Exploring Free Crypto Trading Bots on Reddit: This resource compiles community-driven insights on free trading bots available in the market, giving traders a chance to utilize existing tools.
- The Rise of Trading Bots: Revolutionizing the Financial Markets: This article discusses the impact of trading bots across various financial markets, revolutionizing the way traders participate.
- The Rise of BotTrade: Transforming the Financial Markets: Explore how BotTrade platforms are emerging to change the landscape of automated trading.
Conclusion
In summary, building a trading bot for Binance using Python is both an enriching and educational endeavor. The automation offered by these bots can be transformative in the world of cryptocurrency trading, allowing traders to navigate the complex landscape effortlessly. However, diligent testing and risk management strategies are essential to ensure long-term success.
In my opinion, while using trading bots can provide significant advantages, it’s important to understand the underlying algorithms and market dynamics for optimal results. Successful trading requires a blend of intelligent programming and keen market insight.