How to Make a Crypto Trading Bot in Python
In the burgeoning world of cryptocurrency, trading bots have become indispensable tools for traders seeking to optimize their trading strategies. These automated systems allow for trading on various platforms without needing constant manual input, enhancing efficiency and reducing emotional decision-making. A critical component in the development of these bots is programming, particularly using Python—a versatile language favored in financial technology due to its simplicity and rich libraries. In this comprehensive article, we will guide you through the entire process of creating a cryptocurrency trading bot using Python.
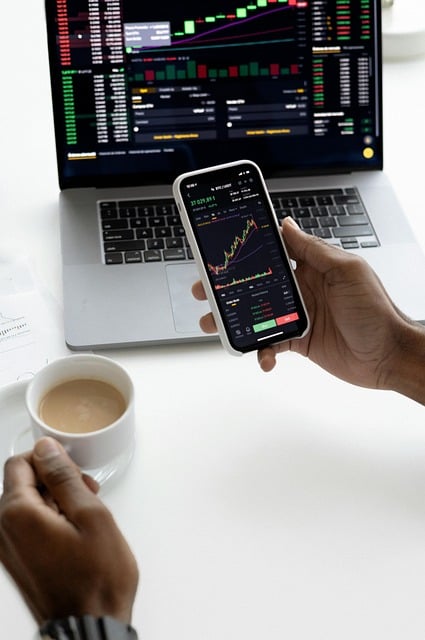
Understanding Cryptocurrency Trading Bots
Before diving into the technical aspects of bot creation, it's imperative to understand what a trading bot is and its functionalities. A trading bot is essentially software programmed to execute trades based on predefined criteria, analyzing market trends, price movements, and other indicators.
The Benefits of Using a Trading Bot
- 24/7 Trading: Unlike human traders, bots can operate continuously, allowing for round-the-clock trading.
- Emotion-Free Trading: Bots follow strict algorithms and are not influenced by emotional responses, which can lead to irrational trading decisions.
- Access to Multiple Exchanges: Bots can be programmed to monitor and trade across various exchanges simultaneously, making price discrepancies easier to exploit.
- Backtesting Capabilities: Bots can analyze historical data to simulate how a trading strategy would have performed in the past.
Setting Up Your Python Environment
Before you can create a trading bot, you need a suitable working environment. This section will take you through the steps to set up Python for your project.
Install Python
First and foremost, ensure that you have the latest version of Python installed on your machine. You can download it from the official Python website.
Install Required Libraries
Once Python is installed, proceed to set up the libraries that your trading bot will require. The most common libraries used for cryptocurrency trading bots include:
- ccxt: A popular library for connecting to cryptocurrency exchanges.
- Pandas: Ideal for data manipulation and analysis.
- NumPy: Useful for numerical computations.
- Matplotlib: Helpful for creating visualizations.
- TA-Lib: Utilized for technical analysis of financial markets.
To install these libraries, simply use pip:
pip install ccxt pandas numpy matplotlib ta-lib
Choosing an Exchange and API Key Setup
Your bot will need access to a cryptocurrency exchange to execute trades. One of the most popular exchanges is Binance, where you can create an account and generate an API key.
Creating an Account on Binance
Visit the Binance website to create your account. Make sure to enable two-factor authentication for added security.
Generating an API Key
After your account is set up, navigate to the API management section. Here, you can create a new API key. Copy the API key and secret, and store them securely.
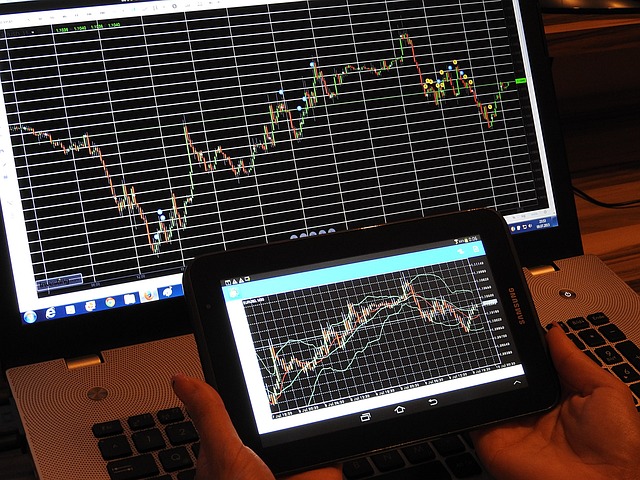
Constructing Your First Trading Bot
With your environment set up and API keys generated, you're ready to build your first trading bot. Below is a simple framework to get you started.
Basic Structure of a Trading Bot
import ccxt
import time
# Set up the exchange
exchange = ccxt.binance({
'apiKey': 'YOUR_API_KEY',
'secret': 'YOUR_API_SECRET',
})
# Define the trading symbol and amount
symbol = 'BTC/USDT'
amount = 0.001
def fetch_price():
return exchange.fetch_ticker(symbol)['last']
def place_order(order_type):
if order_type == 'buy':
order = exchange.create_market_buy_order(symbol, amount)
print(f'Bought {amount} BTC at market price.')
elif order_type == 'sell':
order = exchange.create_market_sell_order(symbol, amount)
print(f'Sold {amount} BTC at market price.')
while True:
current_price = fetch_price()
print(f'Current price of {symbol}: {current_price}')
# Add your trading logic here
time.sleep(60) # Wait for 60 seconds before the next check
Implementing Trading Strategies
Once your bot is operational, it’s crucial to implement a trading strategy that aligns with your investment goals. There are various strategies you can adopt, including arbitrage, trend following, and market-making.
Sample Trading Strategy: Moving Average Crossover
A popular trading strategy involves using moving averages to identify trends. Here’s how to implement a simple moving average crossover strategy:
def moving_average(prices, window):
return sum(prices[-window:]) / window
prices = []
while True:
current_price = fetch_price()
prices.append(current_price)
if len(prices) > 50: # Assuming a short MA of 50
short_ma = moving_average(prices, 50)
long_ma = moving_average(prices, 200)
if short_ma > long_ma:
place_order('buy')
elif short_ma < long_ma:
place_order('sell')
time.sleep(60)
Testing and Backtesting Your Bot
Before deploying your bot in a live trading environment, it's crucial to test it thoroughly. Backtesting on historical data allows you to evaluate how well your strategy would have performed. Use the backtesting capabilities of libraries such as backtrader or QuantConnect.
Simulating Trade Scenarios
You can use historical market data to simulate trade scenarios and refine your strategy accordingly. Adjust parameters, experiment with different strategies, and analyze the outcomes to improve your bot's performance.
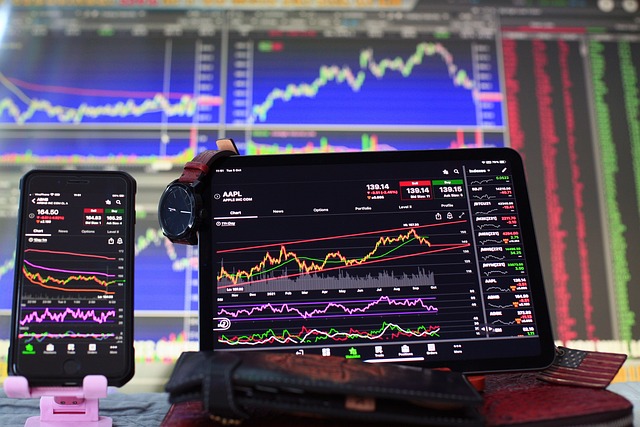
Monitoring Your Bot
Once your trading bot is live, continuous monitoring is essential to ensure performance aligns with expectations. Regularly check for errors, log trades, and adjust settings as required.
Logging and Alerts
Implement logging functionality to keep track of trades and performance metrics. Consider integrating alerts into your bot to notify you of significant changes, trade executions, or other pivotal events.
Staying Informed with Crypto News and Signals
Staying informed about cryptocurrency trends and market signals is crucial. For those interested, the article Unraveling Cryptocurrency Trading Signals: A Comprehensive Guide provides insightful details on interpreting cryptocurrency trading signals to enhance your trading strategy.
For traders focusing on Binance, you may find interest in Binance Bots: Revolutionizing Cryptocurrency Trading, where the discussion revolves around utilizing bots effectively within the Binance ecosystem.
Additionally, the article The Surge of Crypto Telegram: A New Era of Community Engagement in Cryptocurrency highlights the importance of community engagement through Telegram channels, which can provide traders with valuable insights and signals.
Looking towards the future, The Rise of the Binance AI Bot in 2024: A New Era of Cryptocurrency Trading delves into the developments expected in AI-powered bots that could dramatically change trading practices.
Moreover, consider reading The Future of Free Crypto Trading in 2024 to understand the evolving landscape of trading bots and platforms offering free options for traders.
Lastly, ensuring you are equipped with the best tools is crucial. Explore Unraveling the Best AI Crypto Trading Bots in 2023 for insights into the leading bots available in the market today.
Final Thoughts
Creating a crypto trading bot is not just a project for tech-savvy individuals; it’s a valuable initiative for anyone looking to optimize their trading strategies. As you embark on this journey, remember that the market is volatile, and even the best algorithms can fail. Continuous learning, testing, and adaptation are crucial elements for success in cryptocurrency trading.
In conclusion, the principles also discussed in several resources, including the above-mentioned articles, provide crucial insights and a holistic view of the crypto trading landscape. Embrace this innovative technology and refine your strategies to thrive in the dynamic world of cryptocurrency trading.