Creating Your Very Own Crypto Trading Bot with Python
In the rapidly evolving world of cryptocurrency, trading bots have gained immense popularity among traders looking to automate their strategies. If you're a Python enthusiast interested in the crypto market, you're in the right place. In this article, I'll guide you step-by-step on how to create a crypto trading bot using Python, share my insights, and help you build a robust trading solution.
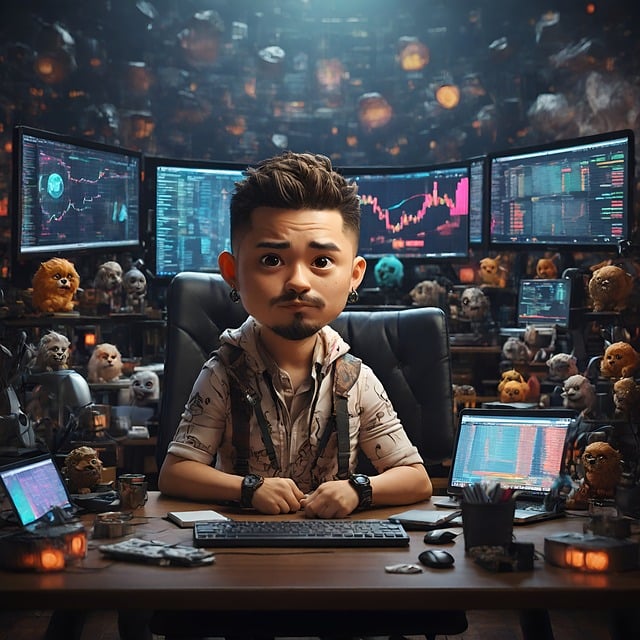
What is a Crypto Trading Bot?
A crypto trading bot is a software program that executes trades on behalf of a trader, based on pre-defined algorithms, market conditions, and trading strategies. These bots can operate around the clock, allowing traders to take advantage of market fluctuations without being glued to their screens.
Why Create Your Own Crypto Trading Bot?
You might wonder why you should create your own crypto trading bot instead of using pre-built options. Here are a few reasons:
- Customization: By building your own bot, you can tailor it to your specific needs and trading strategies.
- Learning Experience: Developing a bot helps you understand both programming and trading, enhancing your skill set in two crucial areas.
- Cost Efficiency: Many pre-built bots come with subscription fees; creating your own could save you money in the long run.
Prerequisites for Building a Crypto Trading Bot
Before diving into the development process, ensure you have the following prerequisites:
- Python Programming Knowledge: Familiarity with Python is essential, as we'll be using it to create the trading bot.
- API Access: You'll need API access to a cryptocurrency exchange for trading operations.
- Market Understanding: A solid grasp of crypto markets and trading strategies will help you design a successful bot.
Setting Up Your Development Environment
Let's start by setting up our development environment:
- Install Python: Make sure you have Python 3.x installed on your system. You can download it from the official Python website.
- Install Required Libraries: Use pip to install the libraries we'll need:
- Create a project directory: Organize your files by creating a new directory for your bot.
pip install ccxt pandas numpy matplotlib
Understanding the API of Your Chosen Exchange
Most cryptocurrency exchanges offer API access, allowing you to interact programmatically with their trading platform. Common exchanges with trading APIs include:
- Binance
- Coinbase Pro
- Kraken
Creating API Keys
To interact with the exchange, you'll need to create API keys:
- Log in to your exchange account.
- Navigate to API management settings.
- Create a new API key and secret. Ensure that you enable trading permissions.
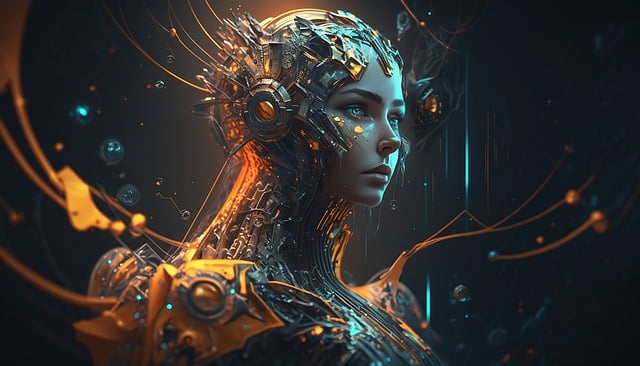
Building the Trading Bot
Step 1: Import Libraries
Open your Python project file and start by importing the necessary libraries:
import ccxt
import pandas as pd
import time
Step 2: Connect to the Exchange
Use your API keys to connect to the exchange:
exchange = ccxt.binance({
'apiKey': 'your_api_key',
'secret': 'your_api_secret',
})
Step 3: Fetch Market Data
Fetching historical price data is crucial for developing your trading strategy:
def fetch_ohlcv(symbol, timeframe='1m', limit=100):
ohlcv = exchange.fetch_ohlcv(symbol, timeframe, limit=limit)
return pd.DataFrame(ohlcv, columns=['timestamp', 'open', 'high', 'low', 'close', 'volume']).set_index('timestamp')
Step 4: Define Your Trading Strategy
This part depends on your trading strategy. For simplicity, let's create a basic moving average crossover strategy:
def simple_moving_average(df, window=20):
return df['close'].rolling(window=window).mean()
Step 5: Implementing the Trading Logic
Now you can create the core logic to execute trades based on the moving averages:
def execute_trade(symbol, order_type, amount):
if order_type == 'buy':
exchange.create_market_buy_order(symbol, amount)
elif order_type == 'sell':
exchange.create_market_sell_order(symbol, amount)
Step 6: Putting It All Together
Now it's time to integrate everything into a main loop:
symbol = 'BTC/USDT'
while True:
df = fetch_ohlcv(symbol)
df['SMA20'] = simple_moving_average(df, 20)
df['SMA50'] = simple_moving_average(df, 50)
if df['SMA20'].iloc[-1] > df['SMA50'].iloc[-1]:
execute_trade(symbol, 'buy', amount=0.001) # Adjust amount as needed
elif df['SMA20'].iloc[-1] < df['SMA50'].iloc[-1]:
execute_trade(symbol, 'sell', amount=0.001) # Adjust amount as needed
time.sleep(60) # Pause for a minute before the next iteration
Testing Your Crypto Trading Bot
Before running your bot with real funds, it's essential to conduct thorough testing. Here’s how:
Backtesting
Use historical data to simulate your bot’s performance. This will give you insights into its efficacy. You can leverage Python libraries such as Backtrader or PyAlgoTrade for this purpose.
Paper Trading
Set up a demo account or use paper trading features that many exchanges offer. This allows you to simulate real trades without financial risk.
Deployment Considerations
Once you've tested and are satisfied with your bot's performance, it’s time to deploy it:
Consider Server Hosting
For uninterrupted performance, consider hosting your bot on a cloud server such as AWS or DigitalOcean, allowing it to run 24/7.
Set Up Monitoring
Implement logging features to keep track of trades made, errors encountered, and overall performance.
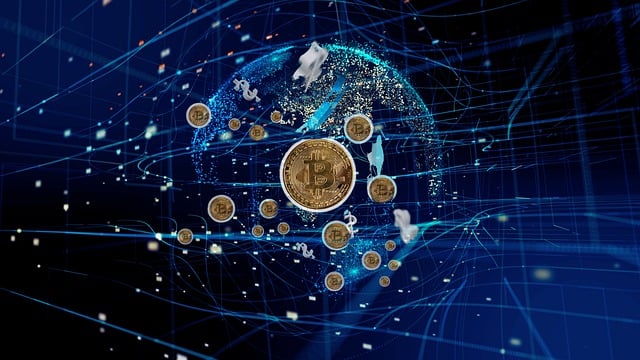
Final Thoughts
Creating a crypto trading bot can be a rewarding experience, combining your passion for coding with the exciting world of cryptocurrency trading. By carefully crafting your bot, following best practices in development, and continuously refining your strategy, you can harness the power of automation in your trading endeavors.
Remember that trading carries risks, and it's essential to invest only what you can afford to lose. Happy coding, and may your crypto trading bot bring you success!