Creating a Trading Bot for Binance Using Python
The cryptocurrency market has exploded over the last decade, giving traders unprecedented opportunities to profit in innovative ways. One of the most dynamic solutions to maximize these opportunities is by creating automated trading bots that can operate on platforms like Binance. This article will explore how to create a trading bot using Python for the Binance exchange, the steps involved, and the considerations essential for successful trading automation.
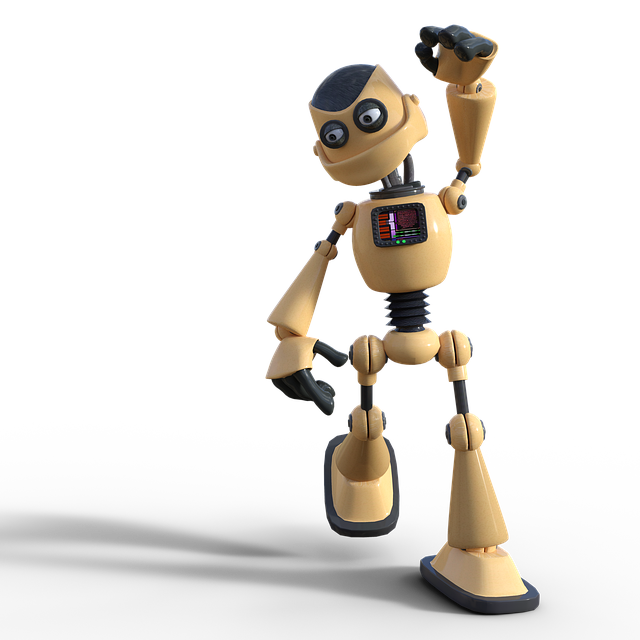
Introduction to Binance Trading Bots
As cryptocurrency trading gains momentum, many traders are looking for ways to automate their strategies. Binance, as one of the largest cryptocurrency exchanges in the world, provides ample opportunities for traders to utilize trading bots. These bots can execute trades on behalf of users based on predefined algorithms, allowing for strategies to be executed without human intervention.
In my opinion, the utilization of trading bots not only streamlines the trading process but also mitigates emotional trading, which can often lead to poor decision-making in volatile markets.
What is a Trading Bot?
A trading bot is software that interacts with financial exchanges (like Binance) to automatically execute trades. These bots can be programmed using different languages – Python being one of the most popular due to its simplicity and wide array of libraries for data analysis and connection to APIs.
Advantages of Using a Trading Bot
- 24/7 Trading: Bots can operate continuously without the need for human supervision.
- Emotionless Trading: Prevents emotional interference that can lead to rash decisions.
- Consistency: Implements your trading strategy without deviation or fatigue.
- Backtesting: Allows traders to test strategies against historical data before deployment.
Essential Tools and Libraries
To create a trading bot for Binance using Python, a few essential tools and libraries will be needed:
1. Python
Ensure you have Python installed on your system. Python 3.6 or higher is recommended for better compatibility with libraries.
2. Binance API
Sign up for a Binance account and enable API access to generate your API key and secret. This is crucial for your bot to communicate with the Binance exchange.
3. Necessary Libraries
- ccxt: A cryptocurrency trading library that facilitates access to numerous exchanges, including Binance.
- Pandas: For data manipulation and analysis.
- NumPy: To perform numerical operations.
- Matplotlib: To visualize data outcomes, if needed.
Steps to Create a Basic Trading Bot
Now, let's delve into the essential steps to create a simple trading bot for Binance.
Step 1: Install Required Libraries
Begin by installing the required libraries using pip:
pip install ccxt pandas numpy matplotlib
Step 2: Connect to Binance
Next, create a new Python file and establish a connection to Binance using your API key and secret:
import ccxt
api_key = 'YOUR_API_KEY'
api_secret = 'YOUR_API_SECRET'
binance = ccxt.binance({
'apiKey': api_key,
'secret': api_secret,
})
Step 3: Fetch Market Data
You need market data to inform your trading strategies. You can fetch OHLCV data (Open, High, Low, Close, Volume) for a specific trading pair:
symbol = 'BTC/USDT'
timeframe = '1h'
limit = 100
data = binance.fetch_ohlcv(symbol, timeframe, limit=limit)
Step 4: Implement a Trading Strategy
Here, you can implement a simple trading strategy. For instance, a moving average crossover strategy could be an excellent start:
import pandas as pd
df = pd.DataFrame(data, columns=['timestamp', 'open', 'high', 'low', 'close', 'volume'])
df['ma_short'] = df['close'].rolling(window=10).mean()
df['ma_long'] = df['close'].rolling(window=30).mean()
if df['ma_short'].iloc[-1] > df['ma_long'].iloc[-1]:
print("Signal: Buy")
else:
print("Signal: Sell")
Step 5: Execute Trades
If your bot generates a buy/sell signal, you can leverage the following code for executing trades:
if df['ma_short'].iloc[-1] > df['ma_long'].iloc[-1]:
binance.create_market_buy_order(symbol, amount) # amount is the quantity you wish to buy
else:
binance.create_market_sell_order(symbol, amount)
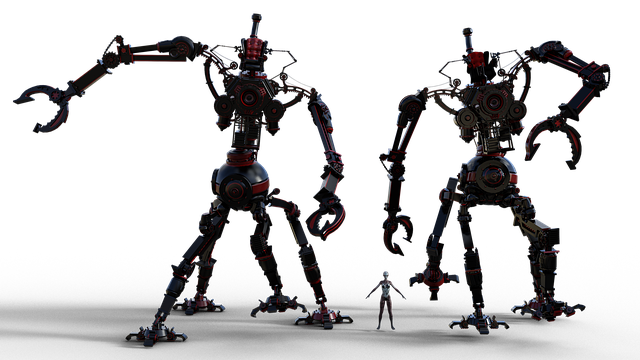
Deployment and Future Considerations
Once you have created the basic bot, consider running it on a virtual private server (VPS) for continuous uptime. Additionally, implementing logging and error handling for bot execution will make it more robust.
In my opinion, it is also imperative to continuously monitor the bot’s performance and adjust your strategies based on market conditions. The cryptocurrency landscape is highly volatile, and what might work today may not necessarily be effective tomorrow.
Resources for Further Learning
If you are interested in a more detailed guide on creating a Binance trading bot, check out Building a Binance Trading Bot in 2024: A Comprehensive Guide. This resource delves deep into the complexities of automated trading, providing the readers with extensive knowledge on various strategies and tools available.
Moreover, for insights on the growing prominence of trading bots in today’s market, I recommend reading Exploring the Rise of Binance Trading Bots in 2024: A Reddit Perspective. It discusses community sentiments and user experiences that revolve around the effectiveness of these bots.
Further, consider exploring the changing landscape of cryptocurrency trading with the article The Rise of Top Crypto Traders: Navigating the Future of Cryptocurrency Investment, which outlines how top-tier traders are leveraging innovative strategies to maintain profitability amidst fluctuating markets.
For those curious about specific trading tools that are gaining traction, The Rise of Sandwich Bot Crypto in 2024: A New Tool for Traders provides insights into this new type of trading bot and its potential applications.
Lastly, to stretch your understanding of automating trading through AI solutions, check out AI Automated Trading Bots: The Future of Investing. This article discusses advancements in artificial intelligence and machine learning and how they are transforming investment strategies in crypto.
Conclusion
Creating a trading bot on Binance using Python can seem daunting at first, but with the right tools and a clear strategy, it can be an exciting venture. As we witness the ever-evolving nature of cryptocurrency trading, utilizing technology to automate and enhance strategies is becoming a staple practice among investors.
In my opinion, as the market matures, the integration of AI and machine learning with trading bots will lead to unprecedented trading efficiencies, making it vital for traders to remain informed and adaptable. The future of trading lies in automation and the smart utilization of data analytics.