Creating a Trading Bot for Binance Using Python
In the rapidly evolving world of cryptocurrency trading, automation has become a game changer. Trading bots, which are automated software programs that execute trades based on predefined algorithms, have surged in popularity among traders. With platforms like Binance offering robust APIs, building your own trading bot in Python can be both a rewarding and educational venture. This guide will walk you through the process of creating a trading bot for Binance using Python, delve into the essential components, explore best practices, and share insights into why this project can be incredibly beneficial.
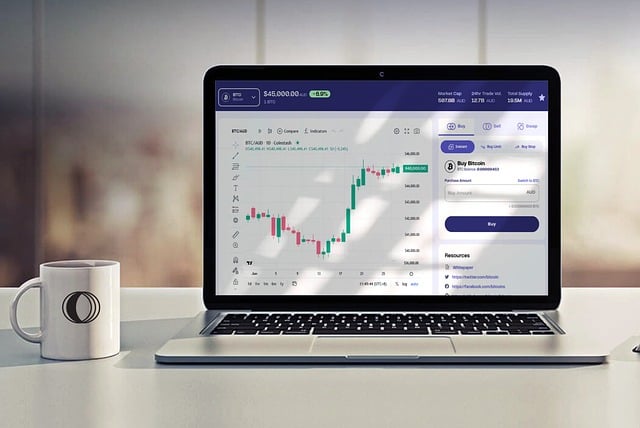
Understanding Binance and Its API
Before diving into the coding aspect, it’s crucial to understand the platform you're working with. Binance is one of the largest cryptocurrency exchanges in the world, known for its wide array of trading pairs and advanced trading features. To harness the power of Binance for your trading bot, you’ll primarily be dealing with its API.
What is an API?
API, or Application Programming Interface, is a set of rules that allows different software entities to communicate with each other. In the context of trading bots, APIs enable you to send trade requests, fetch market data, and manage your account programmatically. Binance offers both a RESTful API and a WebSocket API, which provide real-time data streams that can be critical for responsive trading bots.
Setting Up Your Binance Account
To start trading on Binance, you’ll first need to create an account. After setting up your account, make sure to enable two-factor authentication (2FA) for security. Following this, you’ll need to acquire API keys:
- Log in to your Binance account.
- Navigate to the API Management section of your account.
- Create a new API key, making sure to save your API key and secret securely.
- Set permissions based on whether you want to enable trading, withdrawals, or both.
Prerequisites for Building Your Bot
Having a solid grasp of Python programming and the necessary libraries is key to building a functional trading bot. Additionally, understanding basic trading concepts such as order types (market, limit, stop-loss) is crucial. Here are the main tools and libraries you'll need:
- Python 3 installed on your system.
- The requests library to communicate with the API.
- The pandas library for data manipulation and analysis.
- A library for handling keys, such as python-binance.
Building Your Trading Bot
1. Installing Required Libraries
Start by installing the necessary libraries. You can do this through pip, Python's package installer. Open your terminal and run:
pip install python-binance pandas requests
2. Code Structure
Here’s a simplified structure of what your trading bot code may look like:
from binance.client import Client
import pandas as pd
# Initialize Binance Client
client = Client(api_key='YOUR_API_KEY', api_secret='YOUR_API_SECRET')
def get_price(symbol):
return client.get_symbol_ticker(symbol=symbol)
# Example of fetching price
print(get_price('BTCUSDT'))
Further Developments
You’ll need to add more functionalities such as:
- Market Analysis
- Order Execution
- Error Handling
- Backtesting Strategies
- Logging Results
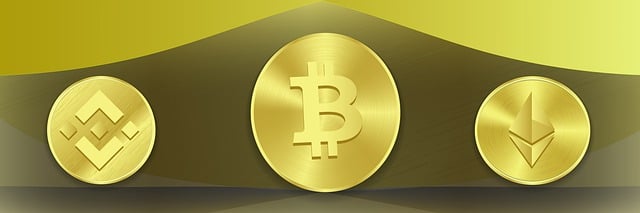
Key Features of a Trading Bot
A successful trading bot should encompass several key features that improve performance and ensure reliability. From my perspective, the following features are paramount:
- Strategy Implementation: Whether you want to follow trends or employ arbitrage strategies, your bot should accurately reflect your trading logic.
- Error Management: Since the cryptocurrency market operates 24/7, your bot should include error handling to manage potential API downtime or changes in market conditions.
- Risk Management: Implementing stop-loss and take-profit orders can help safeguard your investment.
- Performance Monitoring: Keeping track of how your bot is performing over time can offer valuable insights for future adjustments.
Case Studies and Resources
For those looking to expand their knowledge, I highly recommend checking out The Ultimate Guide to 3Comma: Boosting Your Cryptocurrency Trading. This resource dives into enhancing trading strategies using automation tools like 3Commas and explores various trading strategies to maximize profits. It’s enlightening, especially for those seeking effective trading tools in the crypto ecosystem.
Moreover, understanding the broader implications of technology in trading is crucial. The article The Rise of Trading Bots in the Financial Markets elaborates on how trading bots have revolutionized the trading landscape, helping traders to capitalize on opportunities more efficiently. These insights would inspire both new and experienced traders alike.
Finally, when contemplating what to focus on in your trades, the article What to Trade in Crypto: Making the Right Choices provides excellent guidelines on the types of cryptocurrencies worth investing in and the market conditions that favor profitability. It raises critical points about market volatility and how certain coins perform under various circumstances.
Concluding Thoughts
Creating a trading bot for Binance using Python is undoubtedly a fulfilling project that combines technical skills and trading strategy. As a personal observation, the powerful blend of automation and crypto trading has the potential not only to enhance profitability but also to serve as a hands-on learning experience about market dynamics, algorithmic trading, and Python programming.
While building your bot, remember to stay updated on market trends and continuously assess your trading strategies. The landscape of crypto trading is ever-changing, and adaptability is key to sustained success. Happy trading!