Creating a GitHub Binance Trading Bot: A Comprehensive Guide
In recent years, cryptocurrency trading has taken the financial world by storm. As the number of traders increases, so does the demand for automated trading solutions. One popular approach is to use a trading bot to execute trades on exchanges like Binance. In this article, we'll explore how to create a Binance trading bot using GitHub as our primary resource and discuss the essential elements of writing a Binance bot. Whether you’re a complete beginner or a seasoned trader, this guide will help you grasp the fundamentals of trading bots.
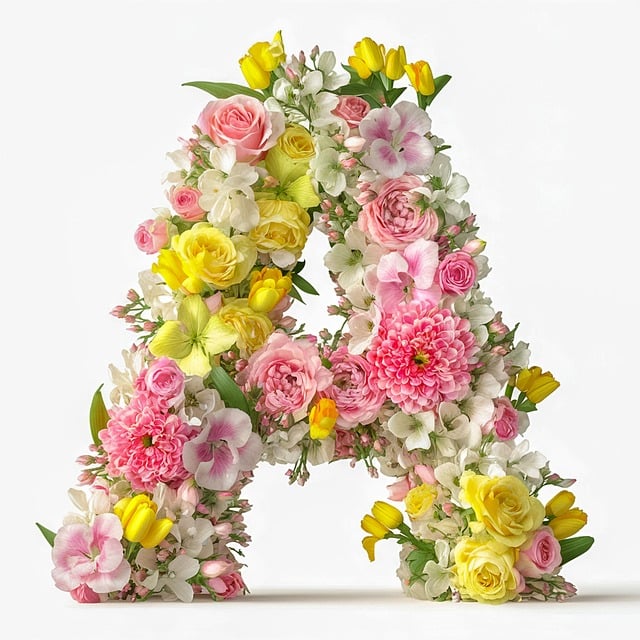
What is a Binance Trading Bot?
A Binance trading bot is a software program that interacts with the Binance exchange, automatically executing buy and sell orders based on predefined strategies and algorithms. These bots can operate 24/7, making it easier for traders to capitalize on market opportunities without constantly monitoring their screens.
Benefits of Using a Trading Bot
- Emotional detachment: bots do not have feelings, which means they can make decisions based purely on logic.
- Time-saving: they work around the clock, allowing traders to focus on other tasks.
- Consistency: bots follow a trading strategy without deviating from it.
- Backtesting capabilities: many bots allow you to test your strategies on historical data.
Getting Started: Setting Up Your Environment
Before diving into writing your Binance trading bot, you need to set up your development environment. Below are the essential steps:
1. Install Python
Most trading bots are written in Python due to its simplicity and extensive libraries. Ensure you have Python installed on your machine. You can download it here.
2. Create a GitHub Account
If you don't already have one, create a GitHub account to use its version control and collaboration features. GitHub is a perfect platform to host your bot code and collaborate with other developers.
3. Set Up a Virtual Environment
Create a virtual environment for your project to keep your dependencies organized. You can do this by running the following commands:
pip install virtualenv
virtualenv myenv
source myenv/bin/activate # On Windows use myenv\Scripts\activate
Connecting to the Binance API
To create your trading bot, you'll need to connect it to the Binance API. This enables your bot to perform various actions, such as retrieving market data and executing trades.
1. Create a Binance Account
If you don't have a Binance account, sign up here. Make sure to complete all verification steps.
2. Generate API Keys
Once your account is set up, navigate to the API Management section in your account dashboard. Create a new API key, ensuring you store the API key and secret securely. Please note: never share your API keys publicly.
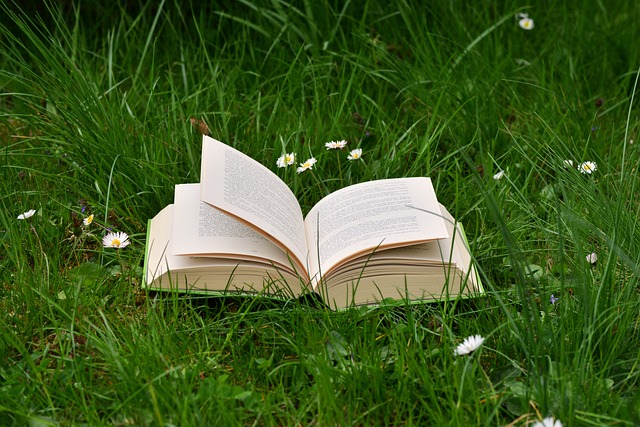
Writing Your Binance Trading Bot
With the environment set up and API keys in place, we can start writing our trading bot. Below are essential components to consider.
1. Setting Up Dependencies
You'll need some external libraries to facilitate interactions with Binance’s API. In your activated virtual environment, install the required libraries:
pip install python-binance numpy pandas
2. Creating the Basic Structure of Your Bot
Create a new Python file named binance_bot.py. Here’s a simple structure to get started:
from binance.client import Client
api_key = 'your_api_key'
api_secret = 'your_api_secret'
client = Client(api_key, api_secret)
# Example function to fetch account balance
def get_account_balance():
balance = client.get_asset_balance(asset='USDT')
return balance
# Fetch and print account balance
print(get_account_balance())
3. Implementing a Trading Strategy
The next step is to implement a trading strategy. Here’s a simple example using a moving average crossover strategy:
import numpy as np
def moving_average(data, window):
return data.rolling(window).mean()
def strategy(symbol, short_window=5, long_window=10):
klines = client.get_historical_klines(symbol, Client.KLINE_INTERVAL_1MINUTE, "1 hour ago UTC")
close_prices = [float(kline[4]) for kline in klines]
data = pd.Series(close_prices)
short_ma = moving_average(data, short_window)
long_ma = moving_average(data, long_window)
if short_ma.iloc[-1] > long_ma.iloc[-1]:
print("Buy Signal")
elif short_ma.iloc[-1] < long_ma.iloc[-1]:
print("Sell Signal")
else:
print("Hold Signal")
strategy('BTCUSDT')
Testing and Backtesting Your Bot
It's crucial to test your trading strategy on historical data to evaluate its effectiveness. Backtesting enables you to simulate trades using historical cryptocurrency price data, assessing how your strategy would have performed.
1. Historical Data Retrieval
You can retrieve historical data using the Binance API. The following snippet demonstrates how to do this:
historical_data = client.get_historical_klines('BTCUSDT', Client.KLINE_INTERVAL_1DAY, "1 Jan, 2021", "1 Jan, 2022")
print(historical_data)
2. Implementing Backtesting Logic
You can extend the previous function to analyze historical data and simulate trades:
def backtest(stock_symbol):
# Implement your backtesting logic here using the historical data
pass
Deployment and Running Your Bot
Once you have created and tested your bot, it's time to deploy it. You can either run your bot locally or deploy it to a cloud server for 24/7 operation. Here are common platforms you can use to deploy your bot:
- Heroku
- AWS (Amazon Web Services)
- Google Cloud Platform
- Vultr
1. Running Your Bot Locally
To run your bot locally, you can simply execute the binance_bot.py script from your terminal:
python binance_bot.py
2. Using a Scheduler for Continuous Operation
To keep your bot running continuously, consider using a task scheduler like cron on Linux or Task Scheduler on Windows, allowing your bot to execute trades at set intervals.
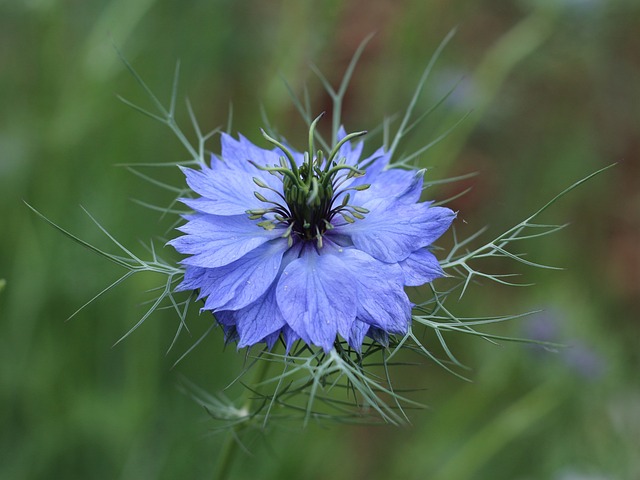
Security Considerations
When dealing with cryptocurrencies, security is paramount. Below are essential security practices:
- Always store your API keys securely.
- Set withdrawal restrictions on your Binance account linked to your trading bot.
- Incorporate error handling within your code to deal with potential API failures or exceptions.
Final Thoughts: Is a Trading Bot Right for You?
Creating a Binance trading bot can be an engaging and potentially profitable venture. However, it’s important to recognize the risks associated with automated trading. Always conduct thorough research and start with small amounts to test your strategies. Automation is not a substitute for experience and market knowledge.
In conclusion, diving into the realm of automated trading through a GitHub Binance trading bot could transform your trading experience. Whether you choose to create your bot from scratch or utilize pre-existing resources on GitHub, the key is to apply sound strategies, diligent testing, and ongoing adjustments to align with market conditions.
Feel free to share your thoughts and experiences in the comments below. Happy trading!