Create Trading Bot on Binance with Python
In the ever-evolving world of cryptocurrency trading, automation is key to maximizing profits and minimizing risks. Trading bots are programs that execute trades based on pre-set conditions, allowing traders to be efficient and strategic. In this article, we will explore creating a trading bot for Binance using Python, examining whether Binance has built-in bots, how to utilize them effectively, and the best practices for both spot and futures trading.
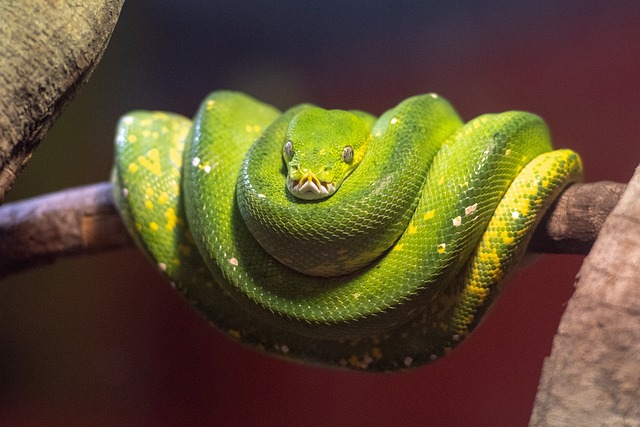
Does Binance Have Bots?
While Binance itself does not provide pre-built trading bots, the platform is robust enough to support the development and deployment of custom trading bots. This functionality allows traders to leverage the Binance API (Application Programming Interface) to fetch market data, execute trades, and manage their accounts programmatically. Many third-party platforms and services offer trading bots that can be integrated with Binance, adding to the flexibility of trading strategies.
How to Use Bots on Binance
To effectively utilize bots on Binance, it’s essential to follow specific steps. Here’s a step-by-step guide for beginners:
1. Create a Binance Account
If you don't already have one, the first step is to register for a Binance account. Ensure that your account is verified and secured with two-factor authentication (2FA) to enhance your security.
2. Generate API Keys
After setting up your account, you’ll need to generate API keys. This allows your bot to securely interact with your Binance account. Navigate to the API Management section of your account settings, create a new API key, and carefully store the key and secret.
3. Choose a Programming Language
Python is a popular choice for creating trading bots due to its simplicity and extensive libraries. Make sure you have a suitable Python environment set up on your local machine.
4. Install Required Libraries
Use pip (Python's package manager) to install the necessary libraries. For Binance, you'll often need libraries like python-binance, which simplifies the API interaction:
pip install python-binance
5. Develop Your Trading Strategy
Before diving into coding, outline your trading strategy. Consider whether you want to focus on day trading, swing trading, or long-term investments. Your strategy will dictate your bot's trading signals, conditions, and risk management rules.
6. Code the Bot
With your strategy in place, start coding your bot. Here’s a simple example to get you started:
from binance.client import Client
api_key = 'your_api_key'
api_secret = 'your_api_secret'
client = Client(api_key, api_secret)
def place_order(symbol, quantity, buy_price):
order = client.order_limit_buy(
symbol=symbol,
quantity=quantity,
price=buy_price
)
return order
# Example usage
order = place_order('BTCUSDT', 0.001, '30000')
print(order)
7. Backtest Your Bot
Once your bot is coded, backtest it with historical data to evaluate its performance. This step is crucial to identify potential flaws in your strategy and refine your approach before deploying it in real-time trading.
8. Monitor and Optimize
After deploying your bot, continuous monitoring is essential. Market conditions change rapidly, and your bot may require adjustments to maintain its effectiveness. Regularly review its performance and optimize your algorithms as necessary.
Binance Crypto Bot: Advantages and Disadvantages
Advantages
- 24/7 Trading: Bots can execute trades around the clock, taking advantage of market fluctuations even when you aren't actively monitoring the market.
- Emotion-Free Trading: Bots follow predefined strategies with no emotional interference, reducing the impact of panic or greed.
- Strategy Implementation: Bots can implement sophisticated strategies that might be challenging for humans to execute manually.
- Data Handling: Bots can analyze vast amounts of market data quickly, making them well-suited for high-frequency trading.
Disadvantages
- Technical Expertise Required: Building a bot requires coding knowledge and a sound understanding of trading strategies.
- Market Volatility: While bots can enhance trading efficiency, they are not immune to market risks, especially in extremely volatile conditions.
- Maintenance: Trading bots require ongoing updates and maintenance to remain effective and aligned with market changes.
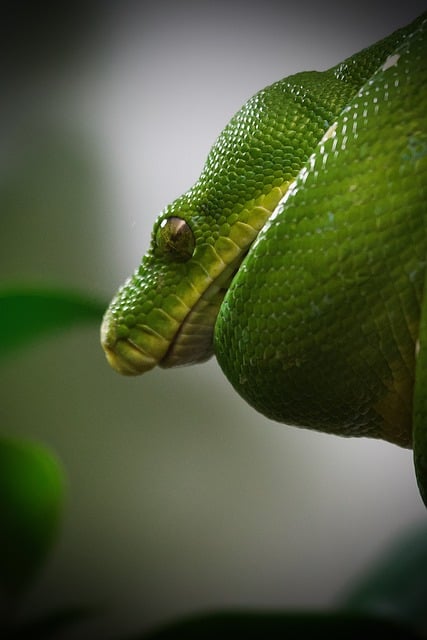
Binance Futures Bot
In addition to its spot trading capabilities, Binance also offers futures trading, which allows traders to speculate on the future price of cryptocurrencies. Futures trading bots can take advantage of leverage and short-selling opportunities.
How to Use a Binance Futures Bot
Utilizing a futures bot involves similar steps to those for spot trading, but there are additional considerations:
1. Understanding Margin and Leverage
Futures trading allows you to use leverage, which can amplify both profits and risks. Make sure you understand how margin works and set your leverage accordingly.
2. Risk Management
In futures trading, implementing strict risk management rules is vital. Ensure your bot includes features such as stop-loss orders and take-profit targets to protect your capital.
3. Advanced Strategies
Futures trading bots may implement advanced strategies such as arbitrage, trend-following, or scalping. Explore different approaches, and backtest them rigorously before applying them in live conditions.
Conclusion
Creating and using a trading bot on Binance with Python is a rewarding venture that can enhance your trading experience. By automating your trading strategies, you can operate more efficiently and make better-informed decisions. As with any investment, be sure to conduct thorough research and maintain a disciplined approach to risk management.
Whether you're interested in spot trading or exploring the world of futures, developing a trading bot can be an excellent way to capitalize on market opportunities while taking control of your trading strategy. Just remember to approach the market with caution and adapt your bot as necessary to stay ahead of the curve.
If you're looking for more resources or inspiration, check out these popular betting tips and sports news websites: