Building a Python Binance Bot: A Comprehensive Guide
In today’s rapidly evolving financial landscape, trading cryptocurrencies has become a multi-billion dollar industry. With the introduction of advanced trading algorithms, many traders seek to automate their trading processes to gain a competitive edge. One of the platforms that have gained immense popularity among traders is Binance. This article delves into the intricacies of building a Python Binance bot, covering everything from setup to advanced trading strategies.
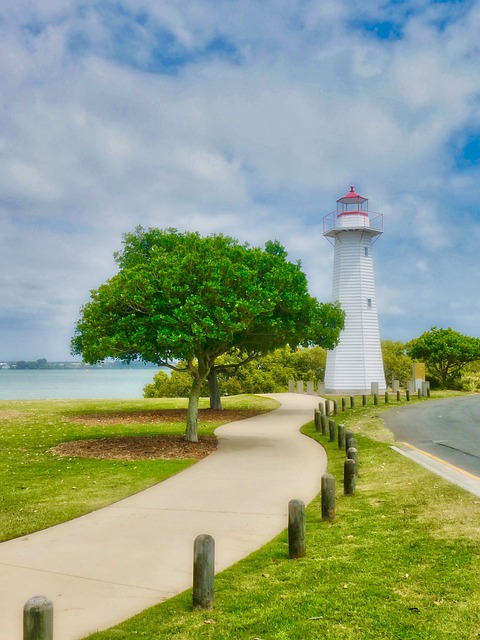
Understanding the Basics: What is a Binance Bot?
A Binance bot is an automated trading program that interacts with the Binance exchange's API (Application Programming Interface) to execute trades. The primary purpose of such bots is to maximize trading profits by analyzing market conditions and executing trades based on predefined strategies.
- Automation: Bots can react to market changes far quicker than humans.
- Backtesting: Allows traders to test strategies on historical data.
- Emotion-free trading: Bots follow algorithms and avoid emotional biases.
- 24/7 Trading: Unlike humans, bots can trade at any hour, increasing opportunities.
The Importance of a Python Binance Bot
Python has emerged as one of the most popular programming languages for developing trading bots, thanks to its simplicity and robust libraries. Using a Python Binance bot can enhance trading efficiency in several ways:
- Access to Automation: Automated trading allows for systematic trading without the need for constant monitoring, which is essential in the volatile cryptocurrency market.
- Ease of Use: Python’s straightforward syntax makes it accessible for both beginner programmers and experienced developers.
- Rich Libraries: Libraries such as 'ccxt', 'pandas', and 'numpy' are particularly beneficial for handling data and implementing complex algorithms.
Setting Up Your Python Environment
Before diving into bot development, setting up the correct environment is crucial. This involves installing Python, pip (Python's package installer), and the necessary libraries to interact with the Binance API.
Installing Python
You can download Python from the official website. Make sure to download the latest version and follow the installation instructions for your operating system.
Verification
Once installed, verify the installation:
$ python --version
$ pip --version
Installing Required Libraries
To interact with the Binance API, install the 'python-binance' library. This can be done using pip:
$ pip install python-binance
Creating Your Binance API Keys
To enable your bot to interact with your Binance account, API keys must be generated. Here’s how to do it:
- Log into your Binance account.
- Navigate to the API Management section.
- Create a new API key and securely save your API and Secret keys.
**Important**: Your API keys grant access to your account. Never share them and ensure that your bot does not expose them in any manner.
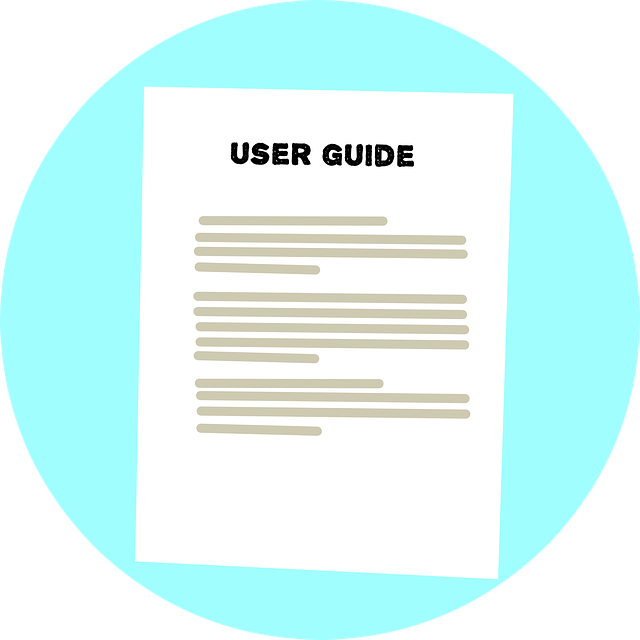
Building Your First Binance Bot
Basic Structure of a Binance Bot
Here’s a simple outline of a Binance bot written in Python. This bot will perform basic operations, such as fetching account data and placing simple orders.
# Import the necessary libraries
from binance.client import Client
# Initialize the Binance client
client = Client(api_key='your_api_key_here', api_secret='your_api_secret_here')
# Check account details
account = client.get_account()
print(account)
# Place a market order (Example: buying 0.01 BTC)
order = client.order_market_buy(
symbol='BTCUSDT',
quantity=0.01
)
print(order)
Understanding the Code
The code above sets up a simple bot that connects to your Binance account and can execute market buying orders. We’ll break down the main components:
- Client Initialization: The bot initializes with your API keys to authenticate with Binance.
- Fetching Account Data: The bot retrieves and prints your account information.
- Placing Orders: The bot uses methods from the Binance API to place trades.
Enhancing Your Bot: Adding Trading Strategies
Once you've built a basic bot, you can implement various trading strategies to enhance its effectiveness. Below are the popular strategies employed in algorithmic trading:
1. Moving Average Crossover
One popular trading strategy is the Moving Average Crossover, where the bot generates buy signals when a short-term moving average crosses above a long-term moving average.
import pandas as pd
# Fetch historical price data
candles = client.get_historical_klines('BTCUSDT', Client.KLINE_INTERVAL_1DAY, '1 year ago UTC')
df = pd.DataFrame(candles, columns=['Open Time', 'Open', 'High', 'Low', 'Close', 'Volume', 'Close Time', 'Quote Asset Volume', 'Number of Trades', 'Taker Buy Base Asset Volume', 'Taker Buy Quote Asset Volume', 'Ignore'])
df['Close'] = df['Close'].astype(float)
# Calculate moving averages
df['Short MA'] = df['Close'].rolling(window=50).mean()
df['Long MA'] = df['Close'].rolling(window=200).mean()
# Generate signals
df['Signal'] = 0
df['Signal'][50:] = np.where(df['Short MA'][50:] > df['Long MA'][50:], 1, 0)
2. MACD (Moving Average Convergence Divergence)
MACD is a trend-following momentum indicator that shows the relationship between two moving averages of a security's price. It can be an excellent addition for traders looking to identify price trend direction.
Implementing MACD
# Calculate MACD
df['EMA12'] = df['Close'].ewm(span=12, adjust=False).mean()
df['EMA26'] = df['Close'].ewm(span=26, adjust=False).mean()
df['MACD'] = df['EMA12'] - df['EMA26']
df['Signal Line'] = df['MACD'].ewm(span=9, adjust=False).mean()
3. RSI (Relative Strength Index)
The RSI is a momentum oscillator that measures the speed and change of price movements, providing signals of overbought or oversold conditions.
# Calculate RSI
def RSI(series, period=14):
delta = series.diff(1)
gain = delta.where(delta > 0, 0)
loss = -delta.where(delta < 0, 0)
avg_gain = gain.rolling(window=period).mean()
avg_loss = loss.rolling(window=period).mean()
rs = avg_gain / avg_loss
rsi = 100 - (100 / (1 + rs))
return rsi
df['RSI'] = RSI(df['Close'])
Backtesting Your Strategies
Backtesting is a critical phase in trading strategy development. It involves testing your trading strategy against historical data to measure its effectiveness. Libraries like 'Backtrader' enable data simulation, helping to assess the performance of your trading strategy.
My OpinionBacktesting is an invaluable tool, as it allows traders to refine their strategies and understand potential weaknesses before risking actual capital. This phase shouldn’t be rushed.
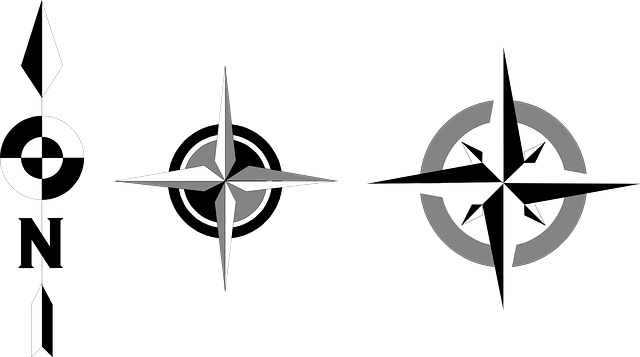
Deploying Your Bot
Once you have tested and refined your bot, it’s time to deploy it. You can run your script locally or use cloud services to ensure your bot operates 24/7 without interruptions.
Choosing Between Local and Server Deployment
- Local Deployment: Suitable for developers who prefer hands-on control but has limitations in uptime.
- Server Deployment: Services like AWS or DigitalOcean provide a more stable environment for your bot to run continuously.
Conclusion: The Future of Trading with Python Binance Bots
The rise of algorithmic trading with tools like Python Binance bots signals a significant shift in how individuals approach market trading. The ability to automate strategies and respond instantaneously to market changes positions algorithmic trading in a dominant space in the investment landscape. However, as with all trading ventures, it is essential to remember that no strategy guarantees success.
In My ViewThe future of trading is undoubtedly intertwined with technological advancement. As more traders leverage tools like Python, the market's dynamics will change, reinforcing the need for continuous education and adaptation. Traders must remain vigilant and open to evolving their strategies in this swiftly moving environment.
As we look forward, anyone interested in cryptocurrencies would benefit significantly from understanding how to build and optimize their trading bots. The growing community around Python and Binance ensures ample resources and support for aspiring and seasoned traders alike.